Git basics: getting started with Git
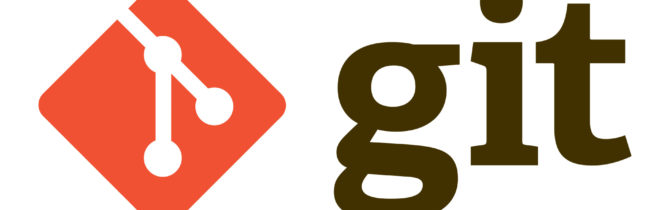
Git is a distributed Version Control System that allows developers to manage their code and maintain bigger projects as the number of the developers involved grow. Today let’s take a look at the basics.
Installing Git
The first thing to do is get the software on your machine:
- On Windows: head here to download it. Once you have installed it you will have an application called “Git Bash” that will take you to a shell. You can also use Cygwin, but it’s more complicated.
- On Mac: head here to download it. Once you have installed, just head to the terminal and you will be able to use git. You can also install it using Brew.
- On Linux: use your package manager to get it.
On Debian-based systems:# apt install git
On RedHat-based systems (replace yum with dnf if you’re using Fedora>21):
# yum install git
First-time configuration
This configuration is needed to know “who you are”. Be careful about the choice, this name and email will be present in every commit you make. Open a terminal and do:
$ git config --global user.name "YOURNAME" $ git config --global user.email [email protected]
Your first repository
Now that you’re ready: fire up your terminal, create a new directory wherever you want, enter that directory and type:
$ git init Initialized empty Git repository in /home/mark/test/.git/
As you can see you’ve just created your first repository! That is confirmed by the .git directory that has been created. That directory contains the whole project and everything that has been committed to git. On the other hand the directory you’re in is called WORKING DIRECTORY.
Adding files
While being in the working directory, create a new file called “file1” and fill it with whatever you feel like. Now type:
$ git add file1
If you typed everything right, you will get no message. In this way you’ve added file1 to the “changes to be committed”. The file isn’t yet inside the git repository.
Committing
You added the file in the precedent step, now it’s time to commit it. While in the working directory do:
$ git commit -m "Initial commit" [master (root-commit) 61ff22c] Initial commit 1 file changed, 1 insertion(+) create mode 100644 file
The -m switch defines the message that describes the commit. Now file1 is tracked inside the repository.
Status
One really helpful command is status. This allows you to see what’s happening inside your working directory. Now, modify file1 and change something, and also create a new file called file2 and fill it with whatever you like. Once done, issue:
$ git status On branch master Changes not staged for commit: (use "git add <file>..." to update what will be committed) (use "git checkout -- <file>..." to discard changes in working directory) modified: file1 Untracked files: (use "git add <file>..." to include in what will be committed) file2
As you can see, the status command shows what is happening in the working directory. Now, do:
$ git add file1 $ git add file2 $ git commit -m "Second commit" [master 3dfc702] Second commit 2 files changed, 2 insertions(+), 1 deletion(-) create mode 100644 file2
In this way you will accept the changes made to file1, add the file2 to repository and commit both.
Removing files
Since we managed to create file2 and add it to repository, now file1 is useless, so we need to remove it:
$ git rm file1 rm 'file1' $ git status On branch master Changes to be committed: (use "git reset HEAD <file>..." to unstage) deleted: file1
As you can see we removed the file1 from the repository. But that doesn’t mean it has been removed entirely. If you check it is still inside the working directory. Now let’s commit the changes:
$ git commit -m "Third commit" [master ea0848a] Third commit 1 file changed, 1 deletion(-) delete mode 100644 file1
Log: a bit of history
Now we’ve done quite the work! But how do we verify each change we’ve made has been recorded? Can we really trust the commit command? We can verify everything we’ve done until now with the log command:
$ git log commit ea0848abbb359c8a294e72785a095e83bc28cd20 Author: mark <[email protected]> Date: Tue Nov 22 12:11:32 2016 +0100 Third commit commit 3dfc7021d6bb957dbd808c4d38e874942d72ce01 Author: mark <[email protected]> Date: Tue Nov 22 12:04:51 2016 +0100 Second commit commit 61ff22ce986db714c34a01bcee0dfe6e56eb098d Author: mark <[email protected]> Date: Tue Nov 22 11:53:00 2016 +0100 Initial commit
As you can see, the log command shows the commits that we have committed to the repository. It also shows many important things about the commits:
- The commit hash: that string after the “commit” keyword identifies each commit unequivocally in the repository.
- The author.
- The date.
- The commit message.
Conclusion
You now know the fundamental commands to move around in a git repository. You know how to add and remove files, view the working directory status, commit changes, and visualize the history of the repository.
- 2020 A year in review for Marksei.com - 30 December 2020
- Red Hat pulls the kill switch on CentOS - 16 December 2020
- OpenZFS 2.0 released: unified ZFS for Linux and BSD - 9 December 2020
Recent Comments